.net core api使用Memory Cache
在ASP.NET CORE
中使用内存缓存还是比较容易的,现在主程序中配置内存缓存
builder.Services.AddMemoryCache( o => {
o.SizeLimit = 100;
});
在控制器中注入MemoryCache
服务
private readonly IMemoryCache _memoryCache;
public CacheRequestController(IMemoryCache memoryCache)
{
_memoryCache = memoryCache;
}
在控制器中实现一个接受路径参数的方法,通过生成多次uuid
以实现一个耗时操作。
[HttpGet("{id}")]
public IActionResult Get(string id)
{
_logger.LogInformation("Request id: {0}", id);
var beforeTime = DateTime.Now;
string? result = _memoryCache.GetOrCreate(id, entry => {
string? uuid = null;
for (int i = 0; i < 2048; ++i) {
uuid = Guid.NewGuid().ToString("N");
}
return uuid;
});
var endTime = DateTime.Now;
var delta = endTime - beforeTime;
return Ok(new { Id = id, Result = result, Time = delta.Microseconds.ToString() });
}
一顿操作猛如虎之后,发生了以下错误
An exception of type 'System.InvalidOperationException' occurred in Microsoft.Extensions.Caching.Memory.dll but was not handled in user code: 'Cache entry must specify a value for Size when SizeLimit is set.'
解决报错的问题:
https://github.com/stefanprodan/AspNetCoreRateLimit/issues/148
根据图中答案进行修改,得到正确运行的代码。
[HttpGet("{id}")]
public IActionResult Get(string id)
{
_logger.LogInformation("Request id: {0}", id);
var beforeTime = DateTime.Now;
string? result = _memoryCache.GetOrCreate(id, entry => {
string? uuid = null;
entry.Priority = CacheItemPriority.High;
entry.Size = 1;
for (int i = 0; i < 2048; ++i) {
uuid = Guid.NewGuid().ToString("N");
}
return uuid;
});
var endTime = DateTime.Now;
var delta = endTime - beforeTime;
return Ok(new { Id = id, Result = result, Time = delta.Microseconds.ToString() });
}
通过结果我们可以看到没有缓存的运行实践大概是在400
毫秒,而获取缓存值大概是在30
毫秒内。
{
"id": "uid",
"result": "8cb3e67bc55e40f3bf9c1b3a097bd7f8",
"time": "408"
}
获取已有缓存的请求结果
{
"id": "uid",
"result": "8cb3e67bc55e40f3bf9c1b3a097bd7f8",
"time": "17"
}
暂无标签
感谢扫码支持
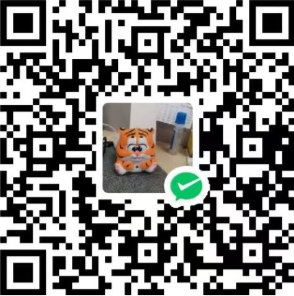
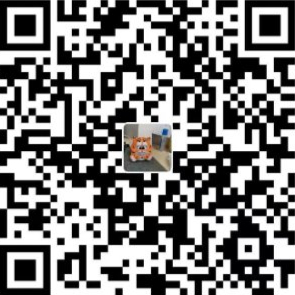